Books
I enjoy writing and I’ve written bunch of books by now. Mostly about R programming, but also on a few other topics.
When I’m working on a book I haven’t sold yet, I usually put the draft on Leanpub where you can get it for free until it is done. Once I’ve sold a book, I have to pull it from Leanpub, but it is a good way to get an early copy, and a good way for me to get feedback while writing. If you get a draft there, then please let me know what you think and how I can improve it.
Below are a complete list of my finished books (plus books that will soon be published).
Future Humans
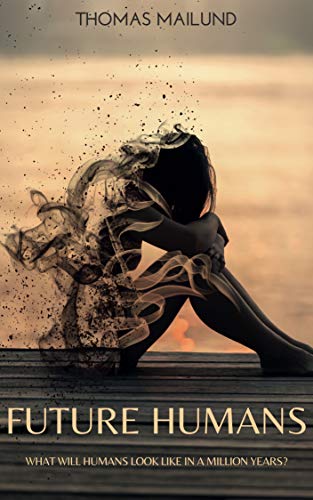
Evolution is driven by random mutations and natural selection. Mutations add variety to a species, and natural selection takes that variety, picks the best and gets rid of the rest, to adapt the species to its environment. We cannot predict where mutations strike, but if we know what they can potentially affect, and we know a species’ environment, then we can attempt to predict where natural selection will go, and how a species might evolve.
We know how the human species evolved over many millions of years, and that it evolved in an environment very different from where we are now. We live in urban areas, our ancestors did not. Medicine gives us a longer and healthier life than we had in our past. We are adapted to one environment, and we now have to adapt to a new one. Where will evolution take us over the next million years?
Shops
Pointers in C programming
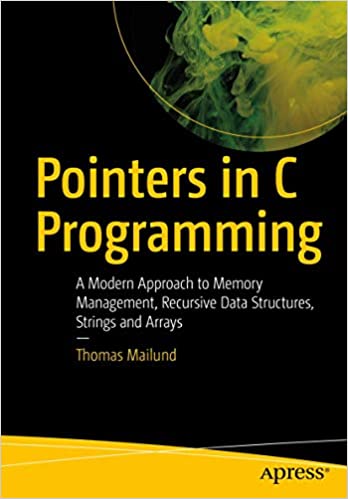
Gain a better understanding of pointers, from the basics of how pointers function at the machine level, to using them for a variety of common and advanced scenarios. This short contemporary guide book on pointers in C programming provides a resource for professionals and advanced students needing in-depth hands-on coverage of pointer basics and advanced features. It includes the latest versions of the C language, C20, C17, and C14.
You’ll see how pointers are used to provide vital C features, such as strings, arrays, higher-order functions and polymorphic data structures. Along the way, you’ll cover how pointers can optimize a program to run faster or use less memory than it would otherwise.
There are plenty of code examples in the book to emulate and adapt to meet your specific needs.
What You Will Learn
- Work effectively with pointers in your C programming
- Learn how to effectively manage dynamic memory
- Program with strings and arrays
- Create recursive data structures
Who This Book Is For
Intermediate to advanced level professional programmers, software developers, and advanced students or researchers. Prior experience with C programming is expected.
Shops
String Algorithms in C
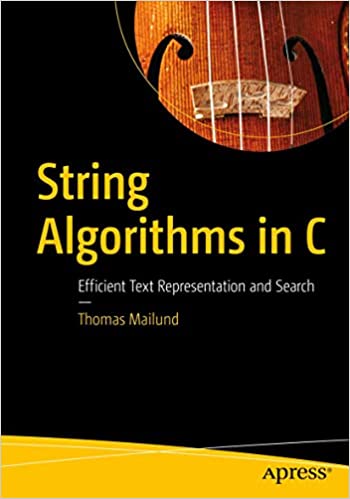
Implement practical data structures and algorithms for text search and discover how it is used inside other larger applications. This unique in-depth guide explains string algorithms using the C programming language. String Algorithms in C teaches you the following algorithms and how to use them: classical exact search algorithms; tries and compact tries; suffix trees and arrays; approximative pattern searches; and more.
In this book, author Thomas Mailund provides a library with all the algorithms and applicable source code that you can use in your own programs. There are implementations of all the algorithms presented in this book so there are plenty of examples.
You’ll understand that string algorithms are used in various applications such as image processing, computer vision, text analytics processing from data science to web applications, information retrieval from databases, network security, and much more.
What You Will Learn
- Use classical exact search algorithms including naive search, borders/border search, Knuth-Morris-Pratt, and Boyer-Moor with or without Horspool
- Search in trees, use tries and compact tries, and work with the Aho-Corasick algorithm
- Process suffix trees including the use and development of McCreight’s algorithm
- Work with suffix arrays including binary searches; sorting naive constructions; suffix tree construction; skew algorithms; and the Borrows-Wheeler transform (BWT)
- Deal with enhanced suffix arrays including longest common prefix (LCP)
- Carry out approximative pattern searches among suffix trees and approximative BWT searches
Who This Book Is For
Those with at least some prior programming experience with C or Assembly and have at least prior experience with programming algorithms.
Shops
String Algorithms in C
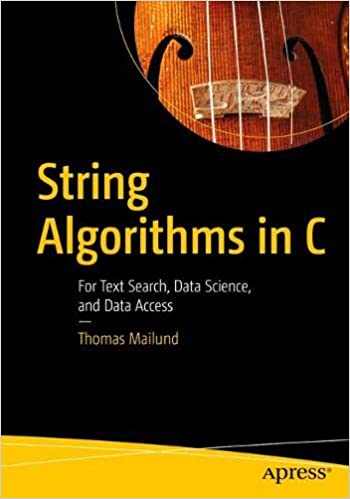
Implement practical data structures and algorithms for text search and discover how it is used inside other larger applications. This unique in-depth guide explains string algorithms using the C programming language. String Algorithms in C teaches you the following algorithms and how to use them: classical exact search algorithms; tries and compact tries; suffix trees and arrays; approximative pattern searches; and more.
In this book, author Thomas Mailund provides a library with all the algorithms and applicable source code that you can use in your own programs. There are implementations of all the algorithms presented in this book so there are plenty of examples.
You’ll understand that string algorithms are used in various applications such as image processing, computer vision, text analytics processing from data science to web applications, information retrieval from databases, network security, and much more.
What You Will Learn
- Use classical exact search algorithms including naive search, borders/border search, Knuth-Morris-Pratt, and Boyer-Moor with or without Horspool
- Search in trees, use tries and compact tries, and work with the Aho-Carasick algorithm
- Process suffix trees including the use and development of McCreight’s algorithm
- Work with suffix arrays including binary searches; sorting naive constructions; suffix tree construction; skew algorithms; and the Borrows-Wheeler transform (BWT)
- Deal with enhanced suffix arrays including longest common prefix (LCP)
- Carry out approximative pattern searches among suffix trees and approximative BWT searches
Who This Book Is For
Those with at least some prior programming experience with C or Assembly and have at least prior experience with programming algorithms.
Shops
Introducing Markdown and Pandoc
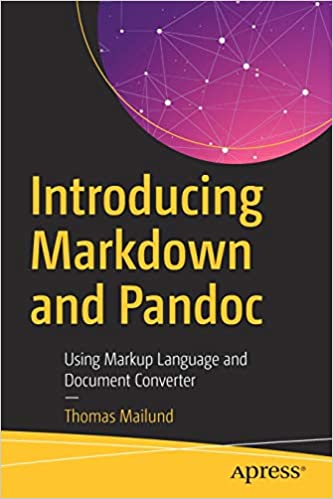
Discover how to write manuscripts in Markdown and translate them with Pandoc into different output formats. You’ll use Markdown to annotate text formatting information with a strong focus on semantic information: you can annotate your text with information about where chapters and sections start, but not how chapter and heading captions should be formatted. As a result, you’ll decouple the structure of a text from how it is visualized and make it easier for you to produce different kinds of output. The same text can easily be formatted as HTML, PDF, or Word documents, with various visual styles, by tools that understand the markup annotations.
Finally, you’ll learn to use Pandoc, a tool for translating between different markup languages, such as LaTeX, HTML, and Markdown. This book will not describe all the functionality that Pandoc provides, but will teach you how to translate Markdown documents, how to customize your documents using templates, and how to extend Pandoc’s functionality using filters. If that is something you are interested in, Introducing Markdown and Pandoc will get you started.
With this set of skills you’ll be able to write more efficiently without worrying about needless formatting and other distractions.
What You Will Learn
- Why and how to use Markdown and Pandoc
- Write Markdown
- Use extensions available in Pandoc and Markdown
- Write math and code blocks
- Use templates and produce documents
Who This Book Is For
Programmers and problem solvers looking for technical documentation solutions.
Shops
R Data Science Quick Reference
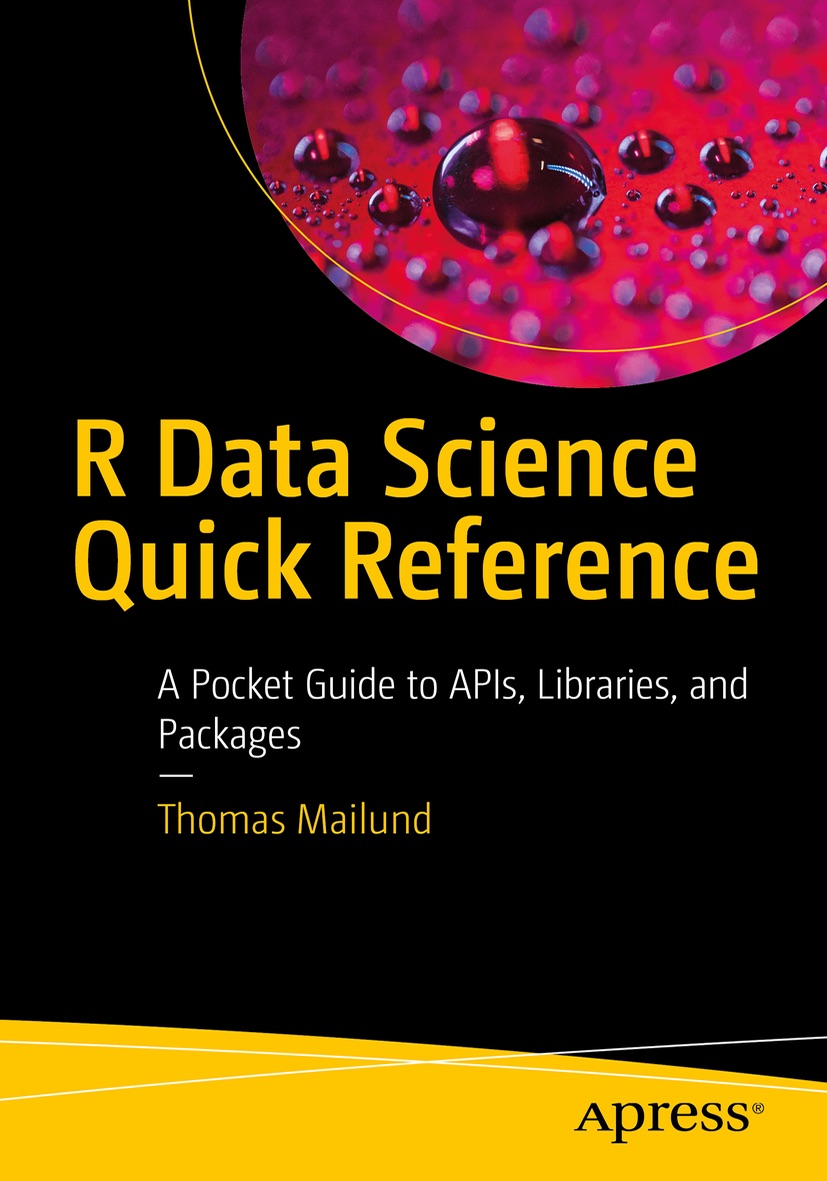
In this handy, practical book you will cover each concept concisely, with many illustrative examples. You’ll be introduced to several R data science packages, with examples of how to use each of them.
In this book, you’ll learn about the following APIs and packages that deal specifically with data science applications: readr, dibble, forecasts, lubridate, stringr, tidyr, magnittr, dplyr, purrr, ggplot2, modelr, and more.
After using this handy quick reference guide, you’ll have the code, APIs, and insights to write data science-based applications in the R programming language. You’ll also be able to carry out data analysis.
What You Will Learn
- Import data with readr
- Work with categories using forcats, time and dates with lubridate, and strings with stringr
- Format data using tidyr and then transform that data using magrittr and dplyr
- Write functions with R for data science, data mining, and analytics-based applications
- Visualize data with ggplot2 and fit data to models using modelr
Who This Book Is For
Programmers new to R’s data science, data mining, and analytics packages. Some prior coding experience with R in general is recommended.
Shops
The Joys of Hashing
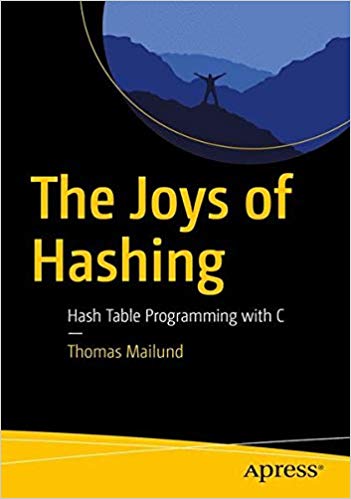
Build working implementations of hash tables, written in the C programming language. This book starts with simple first attempts devoid of collision resolution strategies, and moves through improvements and extensions illustrating different design ideas and approaches, followed by experiments to validate the choices.
Hash tables, when implemented and used appropriately, are exceptionally efficient data structures for representing sets and lookup tables, providing low overhead, constant time, insertion, deletion, and lookup operations.
The Joys of Hashing walks you through the implementation of efficient hash tables and the pros and cons of different design choices when building tables. The source code used in the book is available on GitHub for your re-use and experiments.
What You Will Learn
- Master the basic ideas behind hash tables
- Carry out collision resolution, including strategies for handling collisions and their consequences for performance
- Resize or grow and shrink tables as needed
- Store values by handling when values must be stored with keys to make general sets and maps
Who This Book Is For
Those with at least some prior programming experience, especially in C programming.
Shops
Domain-Specific Languages in R
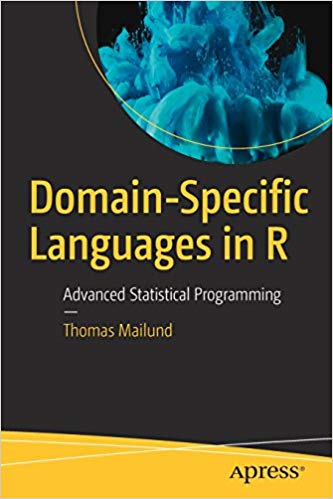
Gain an accelerated introduction to domain-specific languages in R, including coverage of regular expressions. This compact, in-depth book shows you how DSLs are programming languages specialized for a particular purpose, as opposed to general purpose programming languages. Along the way, you’ll learn to specify tasks you want to do in a precise way and achieve programming goals within a domain-specific context.
Domain-Specific Languages in R includes examples of DSLs including large data sets or matrix multiplication; pattern matching DSLs for application in computer vision; and DSLs for continuous time Markov chains and their applications in data science. After reading and using this book, you’ll understand how to write DSLs in R and have skills you can extrapolate to other programming languages.
What You’ll Learn
- Program with domain-specific languages using R
- Discover the components of DSLs
- Implement metaprogramming with DSLs
- Parse and manipulate expressions
Who This Book Is For
Those with prior programming experience. R knowledge is helpful but not required.
Shops
Functional Data Structures in R
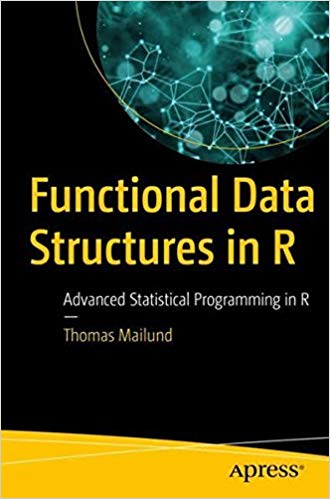
Get an introduction to functional data structures using R and write more effective code and gain performance for your programs. This book teaches you workarounds because data in functional languages is not mutable: for example you’ll learn how to change variable-value bindings by modifying environments, which can be exploited to emulate pointers and implement traditional data structures. You’ll also see how, by abandoning traditional data structures, you can manipulate structures by building new versions rather than modifying them. You’ll discover how these so-called functional data structures are different from the traditional data structures you might know, but are worth understanding to do serious algorithmic programming in a functional language such as R.
By the end of Functional Data Structures in R, you’ll understand the choices to make in order to most effectively work with data structures when you cannot modify the data itself. These techniques are especially applicable for algorithmic development important in big data, finance, and other data science applications.
What You’ll Learn
- Carry out algorithmic programming in R
- Use abstract data structures
- Work with both immutable and persistent data
- Emulate pointers and implement traditional data structures in R
- Build new versions of traditional data structures that are known
Who This Book Is For
Experienced or advanced programmers with at least a comfort level with R. Some experience with data structures recommended.
Shops
Metaprogramming in R
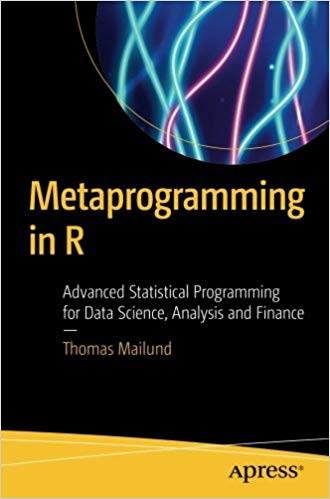
Learn how to manipulate functions and expressions to modify how the R language interprets itself. This book is an introduction to metaprogramming in the R language, so you will write programs to manipulate other programs. Metaprogramming in R shows you how to treat code as data that you can generate, analyze, or modify.
R is a very high-level language where all operations are functions and all functions are data that can be manipulated. This book shows you how to leverage R’s natural flexibility in how function calls and expressions are evaluated, to create small domain-specific languages to extend R within the R language itself.
What You’ll Learn
- Find out about the anatomy of a function in R
- Look inside a function call
- Work with R expressions and environments
- Manipulate expressions in R
- Use substitutions
Who This Book Is For
Those with at least some experience with R and certainly for those with experience in other programming languages.
Shops
Advanced Object-Oriented Programming in R
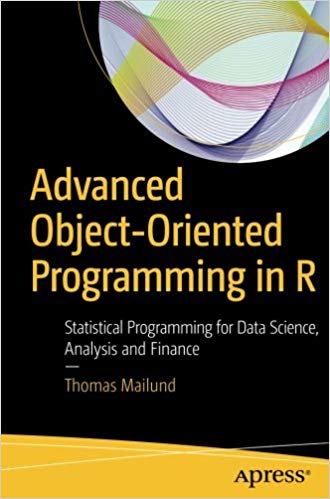
Learn how to write object-oriented programs in R and how to construct classes and class hierarchies in the three object-oriented systems available in R. This book gives an introduction to object-oriented programming in the R programming language and shows you how to use and apply R in an object-oriented manner. You will then be able to use this powerful programming style in your own statistical programming projects to write flexible and extendable software.
After reading Advanced Object-Oriented Programming in R, you’ll come away with a practical project that you can reuse in your own analytics coding endeavors. You’ll then be able to visualize your data as objects that have state and then manipulate those objects with polymorphic or generic methods. Your projects will benefit from the high degree of flexibility provided by polymorphism, where the choice of concrete method to execute depends on the type of data being manipulated.
What You’ll Learn
- Define and use classes and generic functions using R
- Work with the R class hierarchies
- Benefit from implementation reuse
- Handle operator overloading
- Apply the S4 and R6 classes
Who This Book Is For
Experienced programmers and for those with at least some prior experience with R programming language.
Shops
Functional Programming in R
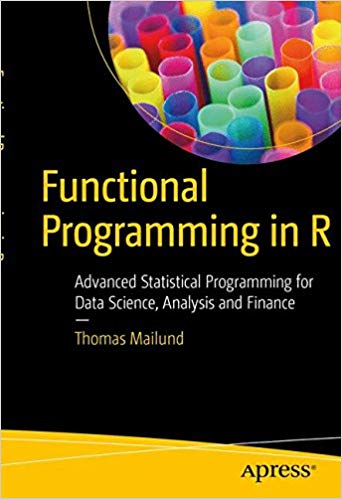
Master functions and discover how to write functional programs in R. In this concise book, you’ll make your functions pure by avoiding side-effects; you’ll write functions that manipulate other functions, and you’ll construct complex functions using simpler functions as building blocks.
In Functional Programming in R, you’ll see how we can replace loops, which can have side-effects, with recursive functions that can more easily avoid them. In addition, the book covers why you shouldn’t use recursion when loops are more efficient and how you can get the best of both worlds.
Functional programming is a style of programming, like object-oriented programming, but one that focuses on data transformations and calculations rather than objects and state. Where in object-oriented programming you model your programs by describing which states an object can be in and how methods will reveal or modify that state, in functional programming you model programs by describing how functions translate input data to output data. Functions themselves are considered to be data you can manipulate and much of the strength of functional programming comes from manipulating functions; that is, building more complex functions by combining simpler functions.
What You’ll Learn
- Write functions in R including infix operators and replacement functions
- Create higher order functions
- Pass functions to other functions and start using functions as data you can manipulate
- Use Filer, Map and Reduce functions to express the intent behind code clearly and safely
- Build new functions from existing functions without necessarily writing any new functions, using point-free programming
- Create functions that carry data along with them
Who This Book Is For
Those with at least some experience with programming in R.
Shops
Beginning Data Science in R
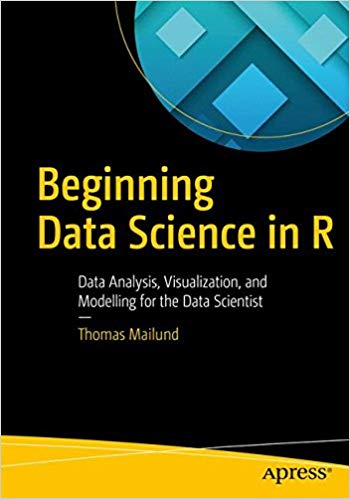
Discover best practices for data analysis and software development in R and start on the path to becoming a fully-fledged data scientist. This book teaches you techniques for both data manipulation and visualization and shows you the best way for developing new software packages for R.
Beginning Data Science in R details how data science is a combination of statistics, computational science, and machine learning. You’ll see how to efficiently structure and mine data to extract useful patterns and build mathematical models. This requires computational methods and programming, and R is an ideal programming language for this.
This book is based on a number of lecture notes for classes the author has taught on data science and statistical programming using the R programming language. Modern data analysis requires computational skills and usually a minimum of programming.
What You Will Learn
- Perform data science and analytics using statistics and the R programming language
- Visualize and explore data, including working with large data sets found in big data
- Build an R package
- Test and check your code
- Practice version control
- Profile and optimize your code
Who This Book Is For
Those with some data science or analytics background, but not necessarily experience with the R programming language.
Shops
The Beginner’s Guide to Markdown and Pandoc
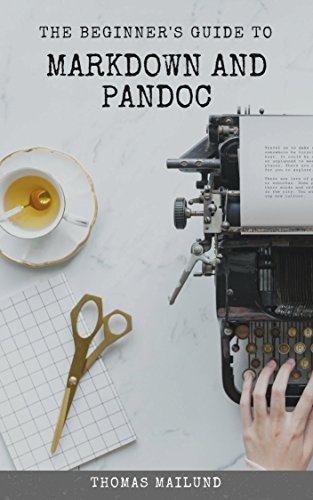
Markdown is a markup language; the name is a pun. Markup languages are used to annotate text formatting information, typically with a stronger focus on semantic information than direct formatting as you would do with WYSIWYG (what you see is what you get) formatting. With markup languages, you might annotate your text with information about where chapters and sections start, but not how chapter and heading captions should be formatted. Doing this decouples the structure of a text from how it is visualised and makes it easier for you to produce different kinds of output. The same text can easily be formatted as HTML, PDF, or Word documents, with various visual styles, by tools that understand the markup annotations.
Pandoc is a tool for translating between different markup languages, such as LaTeX, HTML, and Markdown. I use it for formatting the books I write. This booklet describes how. I will tell you how to write manuscripts in Markdown and translate them with Pandoc into different output formats. I will not describe all the functionality that Pandoc provides, only how it can be used to write papers and books in Markdown. If that is something you are interested in, The Beginner’s Guide to Markdown and Pandoc will get you started.
Shops
There are only e-books of this books because I self-published it and because the print-on-demand option probably isn’t worth the cost. To read it, I would recommend using the Kindle app or a tablet rather than a Kindle; an actual Kindle doesn’t display code particularly nicely.
[ Amazon ] [ iBooks ] [ Leanpub ] [ Gumroad ]
The Beginner’s Guide to Git and GitHub
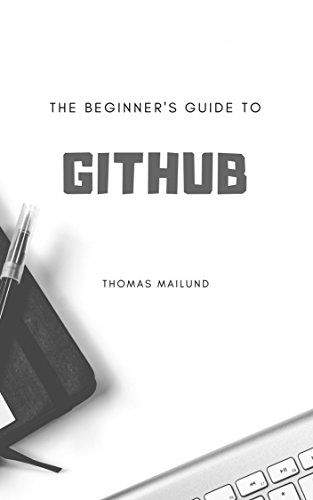
You have heard about git and GitHub and want to know what the buzz is about. That is what I am here to tell you. Or, at least, I am here to give you a quick overview of what you can do with git and GitHub. I won’t be able, in the space here, to give you an exhaustive list of features—in all honesty, I don’t know enough myself to be able to claim expertise with these tools. I am only a frequent user, but I can get you started and give you some pointers for where to learn more. That is what this booklet is for.
Shops
There are only e-books of this books because I self-published it and because the print-on-demand option probably isn’t worth the cost. To read it, I would recommend using the Kindle app or a tablet rather than a Kindle; an actual Kindle doesn’t display code particularly nicely.
[ Amazon ] [ iBooks ] [ Leanpub ] [ Gumroad ]
The Beginner’s Guide to Todoist
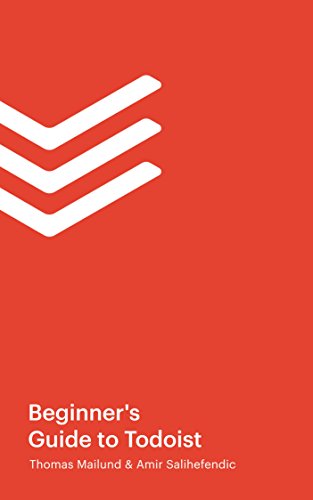
One of the most surprisingly simple tools to increase your productivity and reduce your stress is the todo-list. Too often, we’re overwhelmed with how much we have to do, but by breaking down a complicated project into simpler tasks and then keeping a list of these tasks in the form of a todo-list, you end up getting more done in a calmer way. In fact, as you offload the job of remembering tasks from your active memory to a todo-list, you effectively reduce your stress levels and give yourself more control of your projects and life. This guide describes how to do this using Todoist, the todo-list app that works seamlessly across every device and platform and which has been praised as a life-changing app by The Guardian, USA Today, the New York Times, The Wall Street Journal, Forbes, Lifehacker and more. Inside, you’ll learn the best tips and techniques for using Todoist so you can start living the calmer, more productive and more organized life you’ve always thought you were capable of but never had the chance to achieve.
Shops
There are only e-books of this books because I self-published it and because the print-on-demand option probably isn’t worth the cost. To read it, I would recommend using the Kindle app or a tablet rather than a Kindle; an actual Kindle doesn’t display code particularly nicely.